How Do You Implement Collision Detection in 2D Games?
Collision detection is key in 2D game making. It makes sure characters, objects, and the game world work together smoothly. It checks when things touch or overlap, making games feel real and fun.
Choosing the right algorithm depends on the shapes of the objects. For example, rectangles or circles. Hitboxes, simple shapes around game characters, make it easier to detect collisions.
There are two main steps to add collision detection to 2D games. First, the broad phase uses special data structures to find possible collisions. Then, the narrow phase checks these possibilities with more detailed algorithms. This makes the game run better.
Knowing how collision detection works helps game makers create exciting 2D games. In the next parts, we’ll explore more about how to do this in 2D game development.
Understanding the Fundamentals of Collision Detection
In 2D game development, making collisions work right is key but hard. Games use different ways to check for collisions, like between rectangles and circles. The Axis-Aligned Bounding Box (AABB) is a basic method for checking collisions between shapes that don’t rotate.
Basic Concepts and Terminology
The AABB makes a game object’s shape simple by using a box. This box fits around the object and is aligned with the game’s axes. It makes checking for collisions easy by looking at the boxes, not the shapes themselves.
Types of Collision Detection Systems
- Circle-to-circle collisions are detected by checking if the distance between the centers of the circles is less than the sum of their radii.
- Square-to-square collisions can be detected by comparing the top-left corners and side lengths of the squares.
- For more complex shapes, the Separating Axis Theorem is often used to detect and respond to elastic collisions.
Role of Hitboxes in Game Physics
Hitboxes are key in game physics for collision detection. They are simple shapes that represent game objects. These hitboxes help figure out when and how collisions happen. Knowing about hitboxes is vital for good collision detection in 2D games.
By learning these basics, game makers can make 2D games that are more real and fun. They use Sprite Animation, Level Design, and Game Physics with Axis-Aligned Bounding Box (AABB) collision detection.
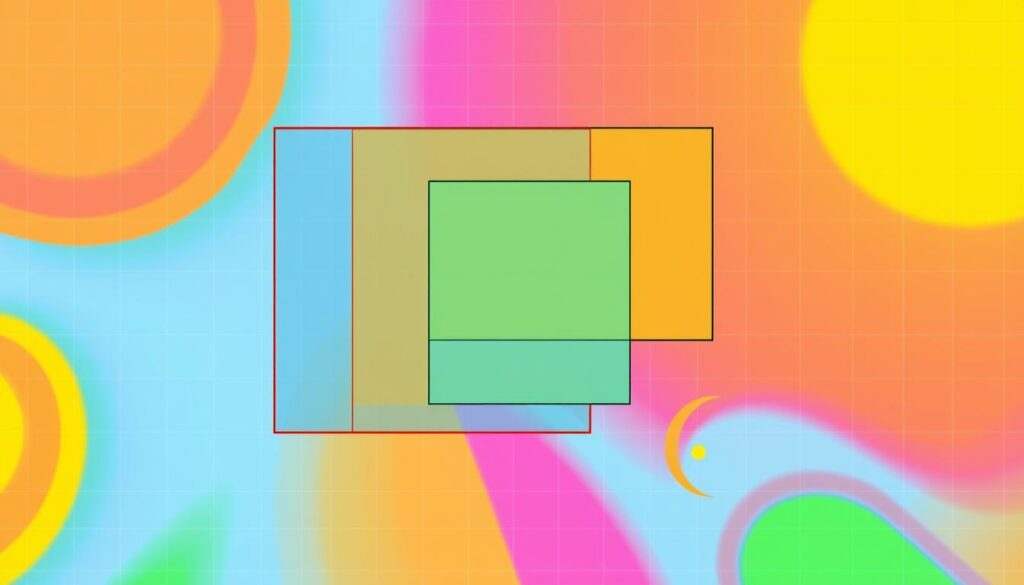
Rectangle Collision Detection in 2D Space
Using Rectangular Hitboxes for collision detection is key in 2D Scripting Languages and Game Testing. It checks if rectangles overlap by looking at their sides. This method is popular for games with rectangular shapes.
The algorithm checks if two rectangles touch by looking at their positions and sizes. It checks four conditions:
- The x-coordinate of rectangle A is less than the x-coordinate of rectangle B plus the width of rectangle B.
- The x-coordinate of rectangle B is less than the x-coordinate of rectangle A plus the width of rectangle A.
- The y-coordinate of rectangle A is less than the y-coordinate of rectangle B plus the height of rectangle B.
- The y-coordinate of rectangle B is less than the y-coordinate of rectangle A plus the height of rectangle A.
If all these conditions are true, the rectangles are colliding. This Rectangular Hitboxes method is simple and fast, making it great for games with many rectangular objects.
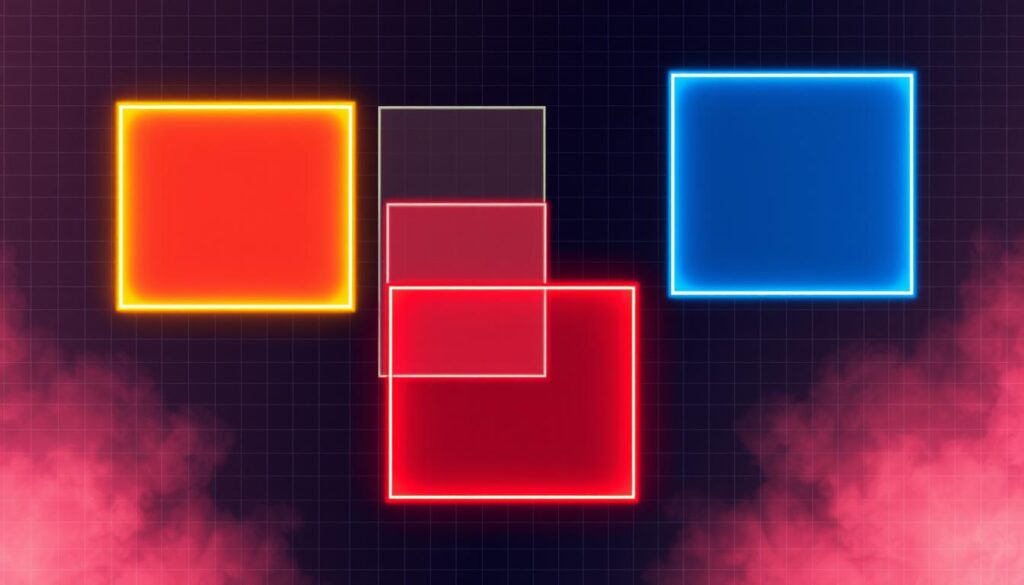
While this method is easy, there are ways to make it better. Using a quadtree or a sort and sweep algorithm can improve performance. These methods are useful for games with lots of objects or complex scenes.
Circle-to-Circle Collision Implementation
In 2D game development, circle-to-circle collision detection is key. It checks if two circles are touching by comparing their distance and size. If they’re too close, a collision is found. This method is great for circles because it’s easy and fast.
Calculating Distance Between Centers
The first step in circle collision detection is finding the distance between their centers. This uses the Pythagorean theorem. It’s like finding the hypotenuse of a right triangle with the circle’s differences in x and y as the legs.
Distance = sqrt((x2 – x1)^2 + (y2 – y1)^2)
Radius-Based Detection Methods
After finding the distance, we compare it to the circles’ sizes. If it’s smaller, the circles are touching. This simple check shows if circles are colliding.
if (Distance
// Collision detected
Optimization Techniques for Circle Collisions
Handling many circle collisions needs a fast system. Using Game Optimization like quadtrees helps a lot. These methods split the game world into parts. This way, only nearby circles are checked, making the system much faster.
Advanced Collision Detection Algorithms
Basic collision detection methods like AABB and circle-to-circle work well for simple 2D games. But for complex shapes and interactions, we need more. The Separating Axis Theorem (SAT) is a powerful tool for detecting collisions between convex polygons.
SAT works by projecting polygons onto axes and checking for overlaps. This method is more accurate and flexible than simpler ones. However, it’s also more complex and requires careful optimization to keep games running smoothly.
These advanced algorithms are key for games with detailed user interfaces and complex shapes. By using SAT and similar techniques, developers can create more realistic and engaging physics. This enhances the player experience, making games more enjoyable for everyone.